Home>Technology and Computers>Noodls x Sentiatechblog Tech Hub
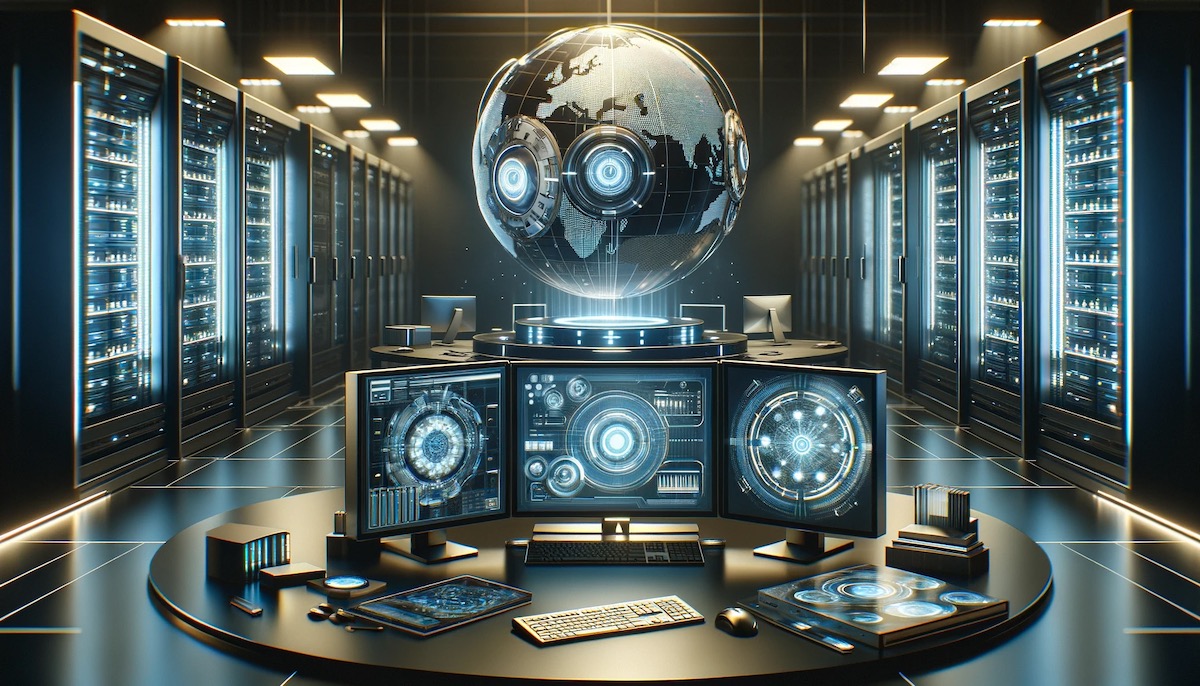
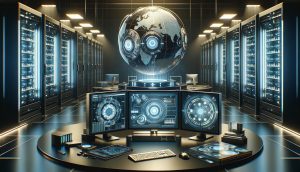
Technology and Computers
Noodls x Sentiatechblog Tech Hub
Published: January 23, 2024
Explore the future of tech with Noodls x Sentiatechblog Tech Hub. Our latest acquisition delivers unparalleled insights and impactful tech content directly to you.
(Many of the links in this article redirect to a specific reviewed product. Your purchase of these products through affiliate links helps to generate commission for Noodls.com, at no extra cost. Learn more)
Table of Contents
Unveiling Tech Innovations
Noodls.com is thrilled to unveil a significant enhancement to our technology and computers section with the introduction of SENTIATECHBLOG, a move underscored by our strategic acquisition of sentiatechblog.com. This acquisition not only expands our footprint in the digital realm but also brings a treasure trove of specialized knowledge and insights directly to our audience. By integrating SENTIATECHBLOG into the Noodls platform, we are bridging the gap between cutting-edge technology news and our readers, reaffirming our commitment to delivering the most relevant and impactful content in the tech industry.
SENTIATECHBLOG on Noodls.com is a testament to our dedication to enriching the user experience with high-quality, in-depth content that spans across a wide array of topics within the technology sector. From the latest in artificial intelligence and machine learning to breakthroughs in software development and cybersecurity, this page will serve as a central hub for tech enthusiasts and professionals alike.Â
As we proudly integrate SENTIATECHBLOG’s wealth of knowledge into Noodls.com, we invite our readers to explore the following articles. This collection of articles not only enriches our existing repository of tech insights but also sets the stage for ongoing exploration and discovery in the ever-evolving world of technology.
-
Aws Re-Invent 2020 Day 3 Optimizing Lambda Cost With Multi Threading -Learn how to optimize Lambda cost with multi-threading at AWS Re-Invent 2020 Day 3. Explore the latest in technology and computers. Unlock cost-saving strategies for your AWS infrastructure.
-
Terraform S3 Cross Region Replication From An Unencrypted Bucket To An Encrypted Bucket -Learn how to set up cross-region replication in Terraform from an unencrypted S3 bucket to an encrypted one. Explore the technology and computer aspects of this process.
-
Aws Cdk Structure Components -Discover the latest technology and computer components with AWS CDK structure. Learn how to build and optimize your infrastructure for maximum efficiency. Explore our comprehensive resources today!
-
Transparently Generate Pre Signed Urls With S3 Object Lambdas -Generate pre-signed URLs with S3 Object Lambdas transparently using advanced technology and computer systems. Simplify access to your S3 objects securely.
-
Geneveproxy An Aws Gateway Load Balancer Reference Application -Explore the latest technology and computer innovations with Geneveproxy, an AWS Gateway Load Balancer reference application. Discover how it can optimize your network performance and security.
-
Ultra Secure Password Storage With Nitropepper -Protect your sensitive data with Nitropepper’s ultra-secure password storage technology. Safeguard your information with advanced encryption and peace of mind. Ideal for technology and computer enthusiasts.
-
Versioning An Asp Net Core Web Api And Publishing It To Azure Api Management -Learn how to version an ASP.NET Core Web API and deploy it to Azure API Management. Get expert tips on technology and computers.
-
Amazon Api Gateway Types Use Cases And Performance -Discover the various types, use cases, and performance of Amazon API Gateway in the realm of technology and computers. Explore its capabilities and benefits for your business.
-
Aws Global Accelerator Terrible Name Awesome Service -Discover how the AWS Global Accelerator, despite its name, delivers exceptional technology and computer services worldwide. Accelerate your digital experience with AWS.
-
Aws Needs To Step Up Its Devops Game And A Few Other Takeaways From The New -Discover the latest insights on technology and computers, including how AWS can enhance its DevOps capabilities and other key takeaways from the new release. Stay ahead in the tech world!
-
A Cost Benefit Analysis Of Vpc Interface Endpoints -Discover the cost benefits of VPC interface endpoints in the realm of technology and computers. Learn how this technology can optimize your network infrastructure.
-
Creating A Custom Webpart For Sharepoint Online Pages -Learn how to create a custom web part for SharePoint Online pages with our comprehensive guide. Explore the latest technology and computer trends.
-
Imposter Syndrome How To Display Front End -Learn how to overcome imposter syndrome and confidently display your front-end technology and computer skills. Discover effective strategies to boost your confidence in the tech industry.
-
Acm For Nitro Enclaves How Secure Are They -Discover the security features of ACM for Nitro Enclaves and learn how they enhance technology and computer protection. Explore the benefits and limitations of this secure technology.
-
Ans Exercise 2 2 Tcpdump Wireshark And Encapsulation -Learn about TCPdump, Wireshark, and encapsulation in this comprehensive guide. Explore the latest technology and computer advancements.
-
Aws Re-Invent 2020 Day 10 The Future Of Cloud Runs On Arm -Discover the latest in technology and computers at AWS Re-Invent 2020 Day 10. Explore how the future of cloud computing is powered by Arm technology.
-
Aws Reinvent 2020 Day 1 S3 Announcements -Discover the latest S3 announcements from AWS Reinvent 2020 Day 1. Stay updated on the newest technology and computer advancements.
-
Bring Your Own Ips To The Aws Cloud Design Considerations -Learn about the design considerations for bringing your own IPs to the AWS cloud. Explore the technology and computer aspects of this migration process.
-
Connecting To A Private Api Gateway Over Vpn Or Vpc Peering -Connect to a private API gateway over VPN or VPC peering for seamless integration with technology and computers. Gain secure access and enhanced connectivity.
-
Acm For Nitro Enclaves Its A Big Deal -Discover how Nitro Enclaves are revolutionizing technology and computers. Learn why ACM considers it a big deal. Explore the impact and potential of this innovative technology.
-
Amazon Vpc The Picasso Of Software Defined Networking -Discover how Amazon VPC revolutionizes software-defined networking. Explore the latest in technology and computer advancements with this innovative solution.
-
Aws Re-Invent 2020 Day 2 Aws Is Coming To A Data Center Or Pizza Parlor Near -Discover the latest in technology and computers at AWS re:Invent 2020 Day 2. Explore how AWS is revolutionizing data centers and pizza parlors near you. Join us for the ultimate tech experience!
-
Aws Transit Gateway For Connecting To On Premise A Thorough Study -Learn how AWS Transit Gateway facilitates seamless connectivity between on-premise data centers and the cloud. Explore the comprehensive study on technology and computers for a deeper understanding.
-
Buckaroo Net Implementation -Implementing Buckaroo Net technology for seamless computer transactions. Enhance efficiency and security with our expert implementation services.
-
Integrating Bitbucket In Aws Codepipeline -Integrate Bitbucket into AWS CodePipeline for seamless technology and computer-based development workflows. Streamline your processes and boost efficiency with this integration.
-
Integrating Eks With Other Aws Services -Integrate EKS seamlessly with various AWS services to optimize your technology and computer infrastructure. Enhance performance and scalability with our expert guidance.
-
Introducing Ftp And Ftps Support For Aws Transfer -Introducing FTP and FTPS support for AWS Transfer. Enhance your technology and computer systems with secure file transfer protocols. Discover the benefits today!
-
Serverless Basic Authentication Using A Custom Authorizer -Learn how to implement serverless basic authentication using a custom authorizer in this comprehensive guide. Explore the latest technology and computer trends.
-
Sql Managed Instance Maintenance -Looking for reliable SQL Managed Instance maintenance services? Our expert team ensures seamless performance for your technology and computer systems. Contact us today!
-
Using Dcevm Hotswap Agent In Java Development -Learn how to use Dcevm Hotswap Agent for seamless code changes in Java development. Stay updated with the latest technology and computer advancements.
-
What I Learned Using The Aws Cdk In The Past Year -Discover valuable insights and lessons learned from using the AWS CDK over the past year. Explore the latest in technology and computer advancements. Gain practical knowledge and tips for leveraging this powerful tool.
-
Why I Love The Cdk -Discover the endless possibilities of technology and computers with the CDK. Explore why this innovative technology is a game-changer for enthusiasts and professionals alike.
-
A Better Way Of Receiving Emails Within End To End Testing -Discover a more efficient method for receiving emails during end-to-end testing. Enhance your technology and computer processes with our innovative solution.
-
A Reactive Java Landscape -Explore the latest trends and advancements in technology and computers with our comprehensive guide to the reactive Java landscape. Stay ahead of the curve with expert insights and analysis.
-
Ans Exercise 2 1 Cisco Csr1000V Nat Instance -Looking for the latest technology and computer solutions? Explore Ans Exercise 2 1 Cisco Csr1000V Nat Instance for cutting-edge insights and innovations.
-
Appsync Insights Part 2 Implementing A Generic String Filter In Python -Learn how to implement a generic string filter in Python with Part 2 of Appsync Insights. Explore the latest in technology and computers.
-
Automated Database Update And Restore With Aws Lambda Functions For Aws -Automate database updates and restores with AWS Lambda functions for AWS. Streamline technology and computer processes with efficient solutions.
- Aws Re-Invent 2020 Day 1 Top 5 Announcements -Discover the top 5 groundbreaking technology and computer announcements from Day 1 of AWS Re-Invent 2020. Stay ahead with the latest innovations and advancements in the tech world.
-
Aws Step Functions The Deployment Orchestrator That Codepipeline Should Have Been -Discover how AWS Step Functions simplifies deployment orchestration, offering the efficiency and control that CodePipeline lacks. Learn more about technology and computers.
-
Debugging A Cdk Python Project In Visual Studio Code -Learn how to debug a CDK Python project in Visual Studio Code with this comprehensive guide. Get expert tips and tricks for efficient debugging. Perfect for technology and computer enthusiasts!
-
Defining A Scheduled Lambda In Cloudformation -Learn how to define a scheduled Lambda function in CloudFormation for efficient automation and management. Explore the technology and computer aspects of scheduling Lambda functions.
-
Deploying A Transit Gateway That Integrates With A Dx Connection On Aws With -Learn how to deploy a transit gateway that seamlessly integrates with a DX connection on AWS. Explore the latest in technology and computer solutions.
-
Features Of A Proper Pipeline Service -Discover the latest technology and computer solutions with our comprehensive pipeline service. Streamline your operations and enhance efficiency today.
-
Introduction To Containers -Learn about the latest technology and computers with our comprehensive introduction to containers. Explore the benefits and applications of this innovative technology.
-
Mediator Pattern In C With Net Core -Learn how to implement the Mediator Pattern in C# with .NET Core. Explore the key concepts and best practices for technology and computers. Gain insights into efficient communication between objects.
-
Re-Invent 2020 Day 4 Top 5 Announcements For Wednesday Friday -Discover the top 5 groundbreaking technology and computer announcements from Re-Invent 2020 Day 4. Stay ahead of the curve with the latest innovations unveiled on Wednesday and Friday.
-
Running Apache In A Docker Container -Learn how to run Apache in a Docker container for efficient technology and computer management. Optimize your workflow with this comprehensive guide.
-
The New Io2 Ebs Volume Type Is Awesome -Discover the amazing features of the new IO2 EBS volume type, revolutionizing technology and computers. Learn how this innovative technology can enhance your computing experience.
-
The Spiraling Complexity Of Dynamodb Data Duplication -Discover the intricacies of DynamoDB data duplication and its impact on technology and computers. Learn how to navigate the spiraling complexity effectively.
-
Use Aws Lambda To Create Ci Cd Dependencies With Codepipeline -Learn how to use AWS Lambda to streamline your CI/CD dependencies with CodePipeline. Explore the latest technology and computer advancements for efficient development workflows.